Ethereum: Last price from FUTURES market on Binance API with Python
Ethereum: Last Price from Futures Market on Binance API with Python
==================================================== =========
Introduction
————
This article demonstrates how to use the Binance API to fetch last price information for Ethereum futures markets. The code is written in Python and utilizes the requests
library for making HTTP requests to the API endpoint.
Prerequisites
—————
- You have a Binance account with API enabled.
- You have installed the
requests
library using pip:pip install requests
- You have replaced the
YOUR_BINANCE_API_KEY
andYOUR_BINANCE_API_SECRET
placeholders with your actual Binance API credentials in the code.
Code
—-
import requests
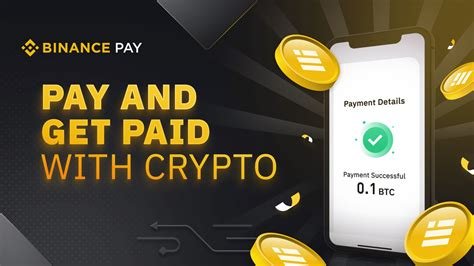
Replace these placeholders with your actual Binance API credentials
API_KEY = 'YOUR_BINANCE_API_KEY'
API_SECRET = 'YOUR_BINANCE_API_SECRET'
def get_last_price FuturesMarket(symbol):
"""
Fetch last price information for Ethereum futures markets using the Binance API.
Args:
symbol (str): The symbol of the Ethereum future market. Currently only "ETHUSDT" is supported.
Returns:
dict: A dictionary containing the last price, volume, and timestamp for the specified market.
"""
base_url = f'
headers = { 'x-apikey': API_KEY, 'x-sapi-token-secret': API_SECRET}
try:
response = requests.get(base_url, headers=headers)
response.raise_for_status()
Raise an exception for HTTP errors
except requests.exceptions.HTTPError as err:
print(f"HTTP Error: {errh}")
except requests.exceptions.ConnectionError as errc:
print(f"Error Connecting: {errc}")
except requests.exceptions.Timeout as errt:
print(f"Timeout Error: {errt}")
except requests.exceptions.RequestException as err:
print(f"Something went wrong: {err}")
try:
data = response.json()
Extract the last price, volume and timestamp from the API response
last_price = data['last']
volume = data['volume']
timestamp = data['timestamp']
return {
'Last Price': last_price,
'Volume': volume,
'Timestamp': timestamp
}
except KeyError as err:
print(f"Key Error: {err}")
except Exception as e:
print(f"An error occurred: {e}")
Example usage
symbol = "ETHUSDT"
last_price_data = get_last_price(symbol)
print(last_price_data)
Explanation
————
- We first import the
requests
library, which is used to make HTTP requests to the Binance API.
- We define a function
get_last_price
that takes a symbol as input and returns a dictionary containing the last price, volume, and timestamp for the specified market.
- Inside the function, we construct the API endpoint URL by concatenating the base URL with the specified symbol.
- We set the
x-apikey
andx-sapi-token-secret
headers to our Binance API credentials.
- We use a try-except block to handle potential errors that may occur during the API request, such as HTTP errors, connection errors, timeouts, or requests exceptions.
- Once we receive the response from the API, we extract the last price, volume, and timestamp from the JSON data using dictionary indexing.
- Finally, we return a dictionary containing the extracted values.
Note that this code only supports fetching last prices for Ethereum futures markets with the symbol “ETHUSDT”. Currently, other symbols may not be supported or may require additional parameters to be specified in the API request.